A Beginner's Guide to Coding Your First Trading Bot: From Concept to Execution
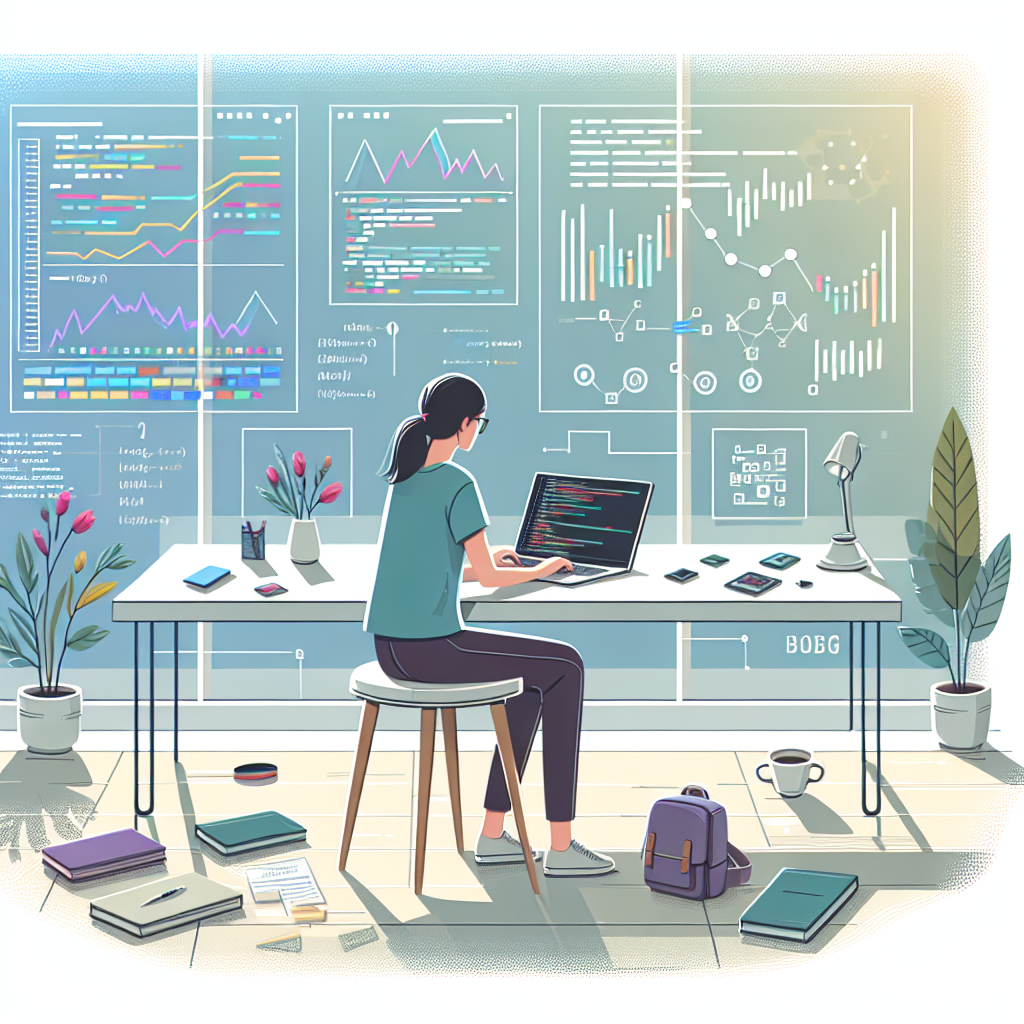
Are you ready to dive into the world of algorithmic trading? Building your first trading bot can be an exciting journey filled with learning opportunities. Whether you’re a beginner coder or a seasoned trader, this guide will take you from the initial concept to executing your very own trading strategy. Let’s break it down step-by-step!
Step 1: Defining Your Strategy
Before jumping into coding, you need a clear trading strategy. This could be based on technical indicators, price action, or even machine learning algorithms. Take some time to research different strategies and choose one that resonates with you. Consider questions like:
- What assets will you trade?
- What time frame will you focus on?
- What indicators or signals will guide your decisions?
Having a well-defined strategy is crucial as it will shape the logic of your bot.
Step 2: Choosing the Right Tools
Selecting the right programming language and tools is vital. Python is an excellent choice for beginners due to its readability and extensive libraries like Pandas, NumPy, and TA-Lib, which simplify data analysis and trading logic implementation. You can also explore platforms like QuantConnect or TradingView, which offer integrated development environments tailored for algorithmic trading.
Step 3: Setting Up Your Development Environment
Once you've chosen your language, it’s time to set up your environment. If you’re using Python, install essential packages using pip:
pip install pandas numpy ta-lib
Next, choose an IDE that suits your style, such as PyCharm, Visual Studio Code, or even Jupyter Notebooks for an interactive experience.
Step 4: Coding Your Trading Bot
Now comes the fun part—coding! Start with a simple structure. Here’s a basic outline of what your bot might look like:
import pandas as pd
import numpy as np
# Load your data
data = pd.read_csv('your_data.csv')
# Define your strategy logic
def simple_moving_average(data, window):
return data['close'].rolling(window=window).mean()
data['SMA'] = simple_moving_average(data, 20)
# Execute trades based on conditions
for i in range(len(data)):
if data['close'][i] > data['SMA'][i]:
print("Buy Signal")
elif data['close'][i] < data['SMA'][i]:
print("Sell Signal")
This code is a simplified example, but it provides a framework for integrating your strategy into a trading bot.
Step 5: Backtesting Your Bot
Before deploying your bot, backtesting is essential. Use historical data to evaluate how your strategy would have performed. This step helps you identify any flaws in your logic and adjust your strategy accordingly. Libraries like Backtrader can be particularly useful here.
Step 6: Live Trading
Once you’re satisfied with your backtest results, it’s time for live trading. Connect your bot to a trading platform via an API (such as Alpaca or Binance). Make sure to start with a small amount to manage risks effectively.
Conclusion
Building your first trading bot is a rewarding experience that combines coding with financial acumen. As you embark on this journey, remember to stay patient and iterative—refine your strategies as you learn. For more resources on algo trading and coding strategies, check out AlgoSamTrader.com. Happy trading, and may your algorithms be ever in your favor!